Booleans! What can you say? They're deceivingly complex features of any language, and only the most proficient among us is capable of using them properly.
Miss M. discovered that one of her cow-orkers found a new way to get the most mileage out of a single boolean variable named count in a single method to see if:
- we're at the end of an enumerator
- send-mail succeeded
- the current job id <= zero
- the length of an exception e-mail is < 2000 characters
- the current job id <= zero (again)
- the current enum enumerator has reached the end of the enum
public override SendMailLog SendEmail(MailInformation mailInfo, JobWorkItems recepients, bool testMode) { var sendMailLog = new SendMailLog(); sendMailLog.SendStart = DateTime.Now; bool count = recepients.Items.Count != 0; if (!count) { throw new ArgumentNullException(" recepients", "Recipient collection is empty, there is no recipients to send to."); } count = !string.IsNullOrEmpty(mailInfo.From); if (!count) { throw new ArgumentNullException( "mailInfo", "Missing from address. SMTP servers do not allow sending without a sender."); } IEnumerator<JobWorkItem> enumerator = recepients.GetEnumerator(); try { while (true) { count = enumerator.MoveNext(); if (!count) { break; } JobWorkItem current = enumerator.Current; var mailMessage = new MailMessage(mailInfo.From, current.EmailAddress); mailMessage.Subject = mailInfo.Subject; mailMessage.Body = PersonalizeEmail(mailInfo, current.EmailAddress); mailMessage.IsBodyHtml = true; try { count = !this.SendMail(mailMessage, testMode); if (!count) { sendMailLog.SuccessMessages.Add(current.EmailAddress); current.Status = JobWorkStatus.Complete; count = current.JobId <= 0; if (!count) { current.Save(); } } } catch (Exception exception) { string str = string.Format("Email: {0}\r\nException: {1}\r\n\r\n", current.EmailAddress, exception.Message); sendMailLog.ErrorMessages.Add(str); current.Status = JobWorkStatus.Failed; count = str.Length < 2000; if (!count) { str = str.Substring(0, 1999); } current.Info = str; count = current.JobId <= 0; if (!count) { current.Save(); } } } } finally { count = enumerator == null; if (!count) { enumerator.Dispose(); } } sendMailLog.SendStop = DateTime.Now; return sendMailLog; }
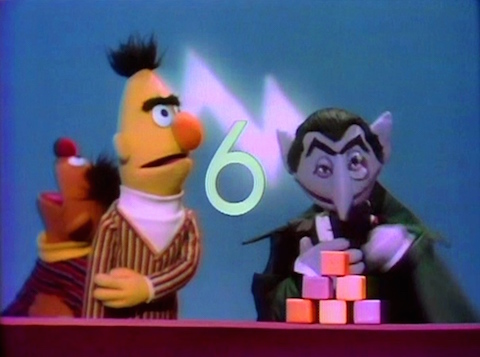
Six! Six reuses of the same variable! AH HA HA HA HA!
